EdgeApp Developers Guide
Linux Development Environment
Although the EdgeApp and CTEC are platform independent applications, the developed source still need to be cross-compiled to the specific target. LMU3040 and LMU3240 are Linux based platforms and thus require Linux based development tools and cross-compiler tool chain. The following sections describe the details for Linux based development.
Getting Started
For Linux development, the following tools are required:
-
A virtual machine or host machine to install the CalAmp ISO
-
An LMU-3x40 and EdgeCore SDK
With the above tools an EdgeApp application can be developed and deployed on the device without writing any code. This process should be attempted first to verify the correct installation of the development tools and is described below.
CalAmp ISO Configuration
CalAmp has taken a Ubuntu 16.04 distribution and installed the required packages to develop EdgeApp. These tools include the EdgeCore SDK as well as Samba, GDB, Eclipse and mayn more developer packages. This section lists some of the configuration details.
- Upgraded Ubuntu packages
- Various packages for development (gcc/g++, gdb, CMake, etc.)
- Latest EdgeCore SDK (/usr/local/oecore-x86_64/)
- Eclipse Neon CDT (/opt/eclipse/)
- Clang static analyzer
- Artistic Style (Source code formatting tool)
- PATH environment variable updated to include the tools above
- Release Notes which includes the version information of the installed packages (Saved in /opt)
- Custom command "isover" and "isover -v" to display the ISO version and release notes respectively
- Python3
- JDK 8
- and many more
The build environment is already installed on the ISO, however the following link outlines the installed information and how to create a custom ISO.
Lastly, you may want to configure Samba.
Configure Samba
# install samba
sudo apt-get update
sudo apt-get install samba
# add a new samba user and set password
sudo smbpasswd -a $USER
# create a share folder
SAMBA_FOLDER=samba_folder
mkdir -p /home/$USER/$SAMBA_FOLDER
# configure samba (smb.conf)
echo "[$SAMBA_FOLDER]" | sudo tee --append /etc/samba/smb.conf
echo "path = /home/$USER/$SAMBA_FOLDER" | sudo tee --append /etc/samba/smb.conf
echo "valid users = $USER" | sudo tee --append /etc/samba/smb.conf
echo "read only = no" | sudo tee --append /etc/samba/smb.conf
# restart samba server
sudo service smbd restart
Virtual Machine
A virtual or host machine is needed to host the CalAmp ISO. This can be customized to fit your needs. However, CalAmp has created simple instructions for setting up a virtual machine from the provided ISO.
Windows
The LMU3040m and LMU2650 are RTOS based platforms and require Windows based tools and cross-compiler tool chain.
For Windows development, the following tools are required:
- Windows 10
- Windows Installer package
- EdgeApp SDK package for Windows
- A LMU3040m or LMU2650
Like the Linux environment, an EdgeApp application can be developed and deployed on the device without writing any code with the tools above. This process should be attempted first to verify the correct installation of the development tools and is described in the next section.
Windows Installer Package
CalAmp has collected the required tools in one zip package that can be found on the CalAmp Developer Portal. Below is a list of the tools included in the package:
- Artistic Style (Source code formatting tool)
- CMake and Graphviz
- Doxygen
- Make
- Python
- Quectel USB Driver
- Snapdragon (Clang tool chain)
- Detailed install instructions
EdgeApp SDK
The EdgeApp SDK version in the package name, such as CTEA_SDK-3.14.0.9.tar.gz, corresponds to the EdgeCore version. The EdgeApp SDK version should match the EdgeCore version running on the LMU3x40.
EdgeApp File System Organization
Directory | Description |
---|---|
CTEA | Contains the EdgeApp documentation start page, edgeapp_documentation.html, a README file, and several supporting files. |
CTEA/deployment | Contains a CMake file to side-load an EdgeApp to a Linux based CTEC device. |
CTEA/doc | Contains the supporting HTML documentation files. |
CTEA/source/components/src/ca_comp | Contains supporting files for CalAmp components to be select-able in the menu config system. |
CTEA/source/components/src/cust_comp | The destination location for new custom development. Also contains the template component, which is recommended to be used to jump-start component development. |
CTEA/source/components/src/ex_comp | Contains source files for components that provide examples on how to accomplish certain tasks. |
CTEA/source/components/src/include | Contains the EdgeApp component hooks header file. These functions must be implemented in each component. Note that using the template component will automatically populate the required functions. |
CTEA/source/components/tool | Contains some supporting files needed in the EdgeApp building process. |
CTEA/source/externals/src/cscripts | Contains an example C-script and template for creating new C-scripts. C-Scripts are for developers interested in converting an existing PEG script to C code, without the need to worry about the component overhead. Note that this does not automatically convert a PEG script. |
CTEA/source/externals/src/ha32_app | Contains a framework to drop in an existing EdgeApp32 (formerly known as HostedApp) and have it work as an EdgeApp without modification. |
CTEA/system/include | Contains EdgeCore API header files. |
CTEA/system/lib | Contains EdgeCore and CalAmp Component libraries specific to the target APP ID. |
CTEA/system/license | Contains license files for CalAmp Component libraries. |
Starting to Program in EdgeApp
Programming an EdgeApp is assisted with the existing template "C" source based application. These files are used to create new components with the basic infrastructure needed for component execution. Once created and installed, this becomes a component that automatically starts, stops, follows the system power states and registers to the message bus for basic system message bus event. The following table outlines the function of all the included starting "C" source.
Source File | Description |
---|---|
include/template_api.h | This is the module public interface document. This contains any defines, types, and public API's. In CTEC, this is the standard definition file for the subsystem. |
src/template.c | This is the main thread/process loop and is the main process. It contains the implementer functions (_impl()) from the public API and the main thread of execution. Additional threads can be launched from the main, refer to the thread example component. This module contains a configurable background loop, access to timers, and event driven implementers. |
src/template.h | This is the main thread header file. |
src/template_api.c | This implements the interface for the component. It contains all the public API that interact with this component within the edgeapp_d daemon via IPC. |
src/template_api_private.h | This is the module private interface document. This contains any defines, types, and private API's. |
src/template_mbus.c | This is the source for interaction with the CTEC message bus and the available channels. Handling of message bus events are performed here. This should be treated as an Interrupt handler and processing should be passed to the main or other threads, where possible, via public or private APIs. |
src/template_mbus.h | This is the is the header file for the template_mbus.c source file. |
src/template_cli.c | This is the Command Line Interface file for the EdgeApp component. This file is used to test the public and private APIs in a development test environment, and in continuous integration automated testing. The existence of the CLI also helps in field debugging. In the Linux environment, it can help to call EdgeApp component public or private API from Linux scripts. Note that command execution occurs in a separate process from the edgeapp_d daemon. |
Refer to Template Component for an in-depth description of how the template component works and what the developer needs to do to customize their own component.
The SDK comes with a CMake process with the following options:
- make - compiles the existing source.
- make deploy - compiles the existing source, packages the build artifacts for download to the target, and deploys them to an attached target.
- make style - runs Astyle on the "C" source for code beautification.
- See Building EdgeApp for steps on building an EdgeApp and running the static analyzer.
CalAmp Telematics EdgeCore Development Environment
CTEC Basic Architecture
CTEC Module Descriptions
All of the EdgeCore modules have a public interface that allow for the control, configuration, and queries of the internal operations of the application. These are documented fully in the CTEC doxygen-based high-level and detailed design documentation. The following table gives a general description to help guide through this documentation.
CTEC Subsystem | Description of Subsystem |
---|---|
ATCMD_API | The AT Command subsystem provides a programmatic call the AT Command parser. This subsystem has a library containing the public interface, a daemon process and threads, and a CLI for testing all API's. |
BLE_API | This is the Bluetooth Low Energy subsystem and contains the public API's associated with a variety of BLE roles available. This subsystem has a library containing the public interface, a daemon process and threads. |
CMN_API | This is a common utility module. This contains general purpose functions that are self contained to the CTEC platform. There are no OS or System calls in this subsystem, This subsystem is only a library containing the public interface. |
CONNMGR_API | Connection manager is responsible for maintaining the state of both Broadband and local connections. It controls the connected state of MDMMGR, WiFi, and BLE. For connection management, the Connection Manager API should be used over the API in these aforementioned subsystems. This subsystem has a library containing the public interface, a daemon process and threads. |
EDGEAPP_API | This is the EdgeApp process that connects the EdgeApp components to CTEC. This subsystem has a library containing the public interface, a daemon process and threads, and has a CLI for testing all API's. |
FRAMEWORK | The framework subsystem is the class structure of all CTEC and CTEA applications. It represents the tasking structure, the IPC model, and provides the base functionality of all process as well as file system APIs. It utilizes the OSA/POSIX abstraction layers to provide compatibility. It is recommended to use the CTEC file system over generic OS API to be compatible over multiple CTEC Platforms. This subsystem is a library only. |
GPS_API | The GPS subsystem contains the API's required for enabling GPS services and getting both position and GPS time information. The APIs generate position report events, as well as message bus events. This subsystem has a library containing the public interface, a daemon process and threads. |
IGNMGR_API | This is the ignition manager module. It provides an interface to monitor the ignition state. This subsystem has a library containing the public interface, a daemon process and threads. |
IO_API | This is the I/O module. Real and Virtual inputs can be read and events can be subscribed to. This subsystem has a library containing the public interface, a daemon process and threads. |
LMDIRECT_API | The Location Message (LM) Direct subsystem contains the API to generate LM Direct messages. These messages would be destined to or received from the inbound servers that the device is connected to in config. This subsystem has a library containing the public interface, a daemon process and threads. |
MBUS_API | CTEC has a proprietary message bus used for general IPC traffic. Useful for event generation, but can also be use to read existing channel and register for events. Custom messages bus may also be created. This subsystem has a library containing the public interface, a daemon process and threads. |
MOTIONMGR_API | Motion manager managers general configuration of the MEMS device, as well as driver behavior and motion logs. This subsystem has a library containing the public interface, a daemon process and threads |
PARAM_API | The parameter subsystem is a fully self contained non-volatile (NV) storage for configuration parameters and s-registers. In addition to the param_api.h for parameter configuration, see also config_init_param.h and param_id_defs.h for parameter definitions and sreg_id_defs.h for s-register definitions. It is recommended to use the CTEC NV storage over generic OS API to be compatible over multiple CTEC Platforms. This subsystem has a library containing the public interface only. |
PEG_API | This is the PEG2 subsystem. This subsystem has a library containing the public interface, a daemon process and threads. |
PERIPHDRV_API | Provides access to battery status, ADC, GPIO and other peripheral devices. This subsystem has a library containing the public interface, a daemon process and threads. |
PSM_API | SEE IMAGE BELOW This is the Power State Machine module. This is responsible for sleep operations, and system board state. This subsystem has a library containing the public interface, a daemon process and threads. |
SERIAL_API | The Serial API is a serial interface API allowing the connection of virtual serial devices to real serial devices. This is accomplished by configuration parameters that can map a given UART say to a give process. There are a number of usages of this, one example of this being an external device may be physically connected to a BLE SPS interface, or a physical UART. The application can switch between each via config parameters and not changing code. This subsystem is only a library containing the public interface |
SYSTIME_API | System Time API. Indicates current times source and selection of time source. This subsystem has a library containing the public interface, a daemon process and threads. |
VBUSDRV_API | Vehicle bus interface API. This subsystem has a library containing the public interface, a daemon process and threads. |
WDOG_API | Watchdog subsystem monitors all CTEC and CTEA threads of execution to detect subsytem malfunctions. This subsystem has a library containing the public interface, a daemon process and threads. |
WIFIMGR_API | Wifi is available on the LMU3240, this subsystem configures the control of common WiFi functions. This subsystem has a library containing the public interface, a daemon process and threads. |
ZONE_API | This subsystem contains CalAmps Zones and Geo-Zones applications. This subsystem has a library containing the public interface, a daemon process and threads. |
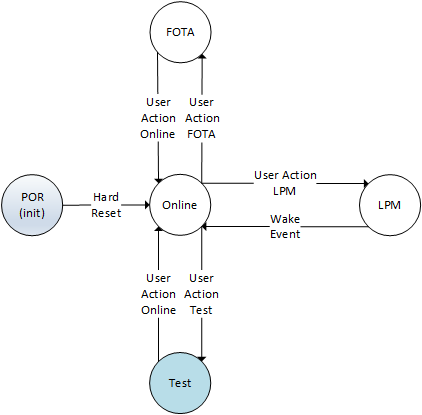
PSM_API
Updated about 1 year ago